Newping.h is a Library developed by Tim Eckel. It makes interfacing and using ultrasonic sensors much easier. The library can be installed directly from the Arduino IDE, or the zip file can be downloaded from GitHub. The default method of using an ultrasonic sensor was difficult and didn’t give much flexibility, with this library many can be achieved easily.
Using this library, we can connect the echo and trigger pins to a single digital pin on a microcontroller. This single-line communication makes interfacing multiple sensors easy.
How to install the Newping.h library
Install the library by going to manage library and searching for newping. Install the library by Tim Eckel.
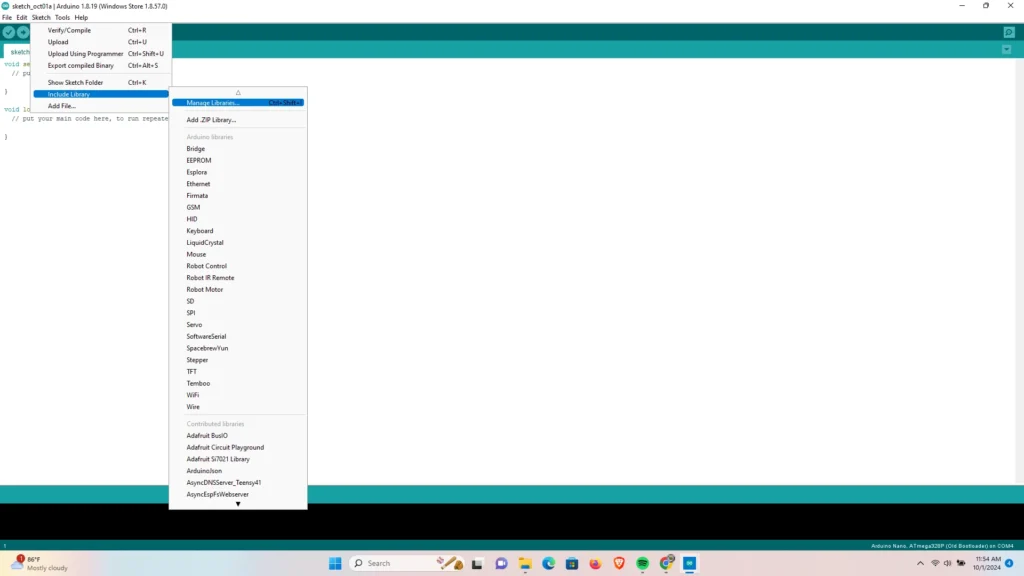
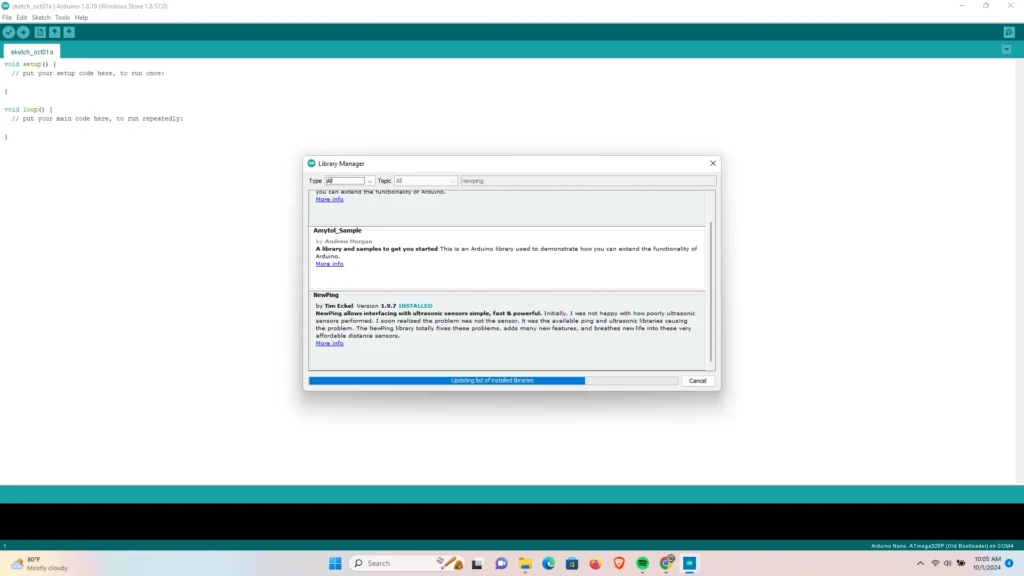
Using the Library for projects
Two-line connection with ultrasonic sensor.
Use the code and circuit diagram below. In this example, we connected the trigger to pin 10 of the Arduino and Echo to pin 11.
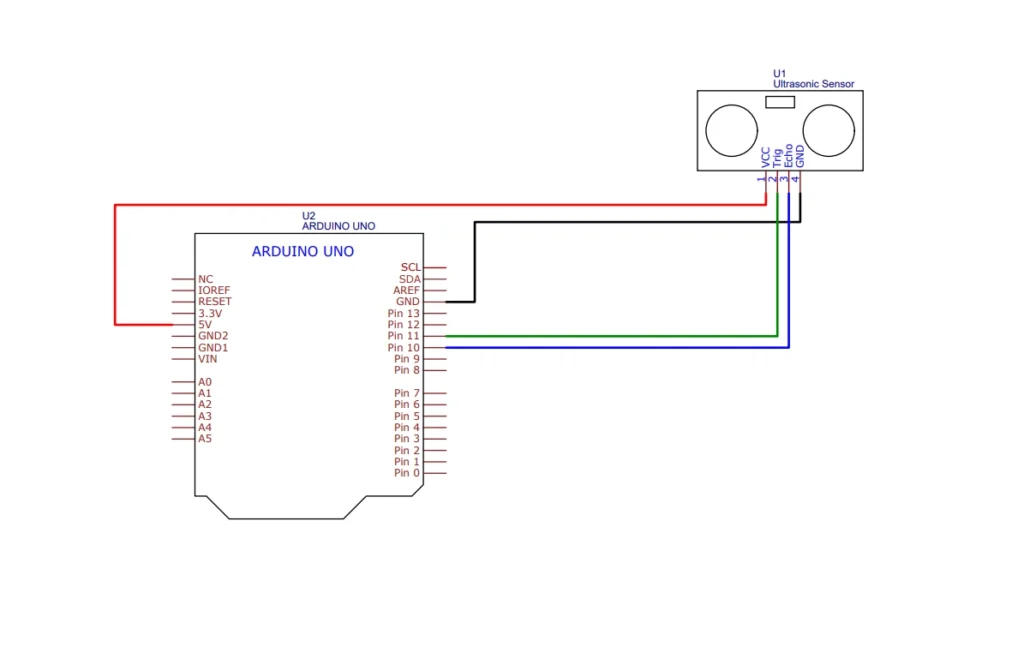
#include <NewPing.h>
#define TRIGGER_PIN 10 // Arduino pin tied to trigger pin on the ultrasonic sensor.
#define ECHO_PIN 11 // Arduino pin tied to echo pin on the ultrasonic sensor.
#define MAX_DISTANCE 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm.
NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // NewPing setup of pins and maximum distance.
void setup() {
Serial.begin(9600); // Open serial monitor at 115200 baud to see ping results.
}
void loop() {
delay(50); // Wait 50ms between pings (about 20 pings/sec). 29ms should be the shortest delay between pings.
Serial.print("Ping: ");
Serial.print(sonar.ping_cm()); // Send ping, get distance in cm and print result (0 = outside set distance range)
Serial.println("cm");
}
The above code will give you the following result in the serial monitor.
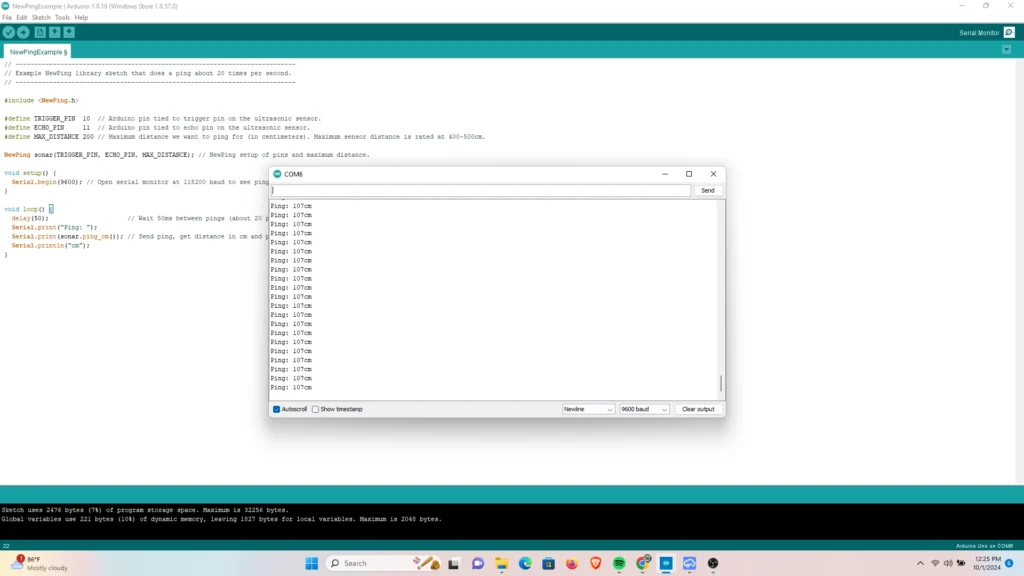
One-line connection with ultrasonic sensor
In this example, we connected the echo and trigger pins to pin 10 of the Arduino. follow the circuit diagram and code supplied below.
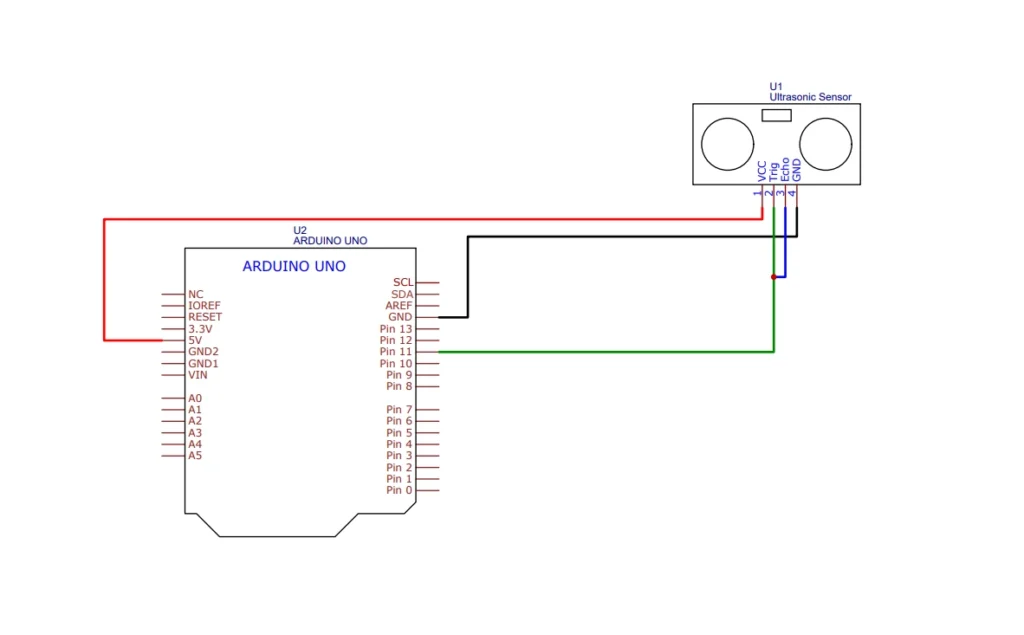
#include <NewPing.h>
#define TRIGGER_PIN 10 // Arduino pin tied to trigger pin on the ultrasonic sensor.
#define ECHO_PIN 10 // Arduino pin tied to echo pin on the ultrasonic sensor.
#define MAX_DISTANCE 200 // Maximum distance we want to ping for (in centimeters). Maximum sensor distance is rated at 400-500cm.
NewPing sonar(TRIGGER_PIN, ECHO_PIN, MAX_DISTANCE); // NewPing setup of pins and maximum distance.
void setup() {
Serial.begin(9600); // Open serial monitor at 115200 baud to see ping results.
}
void loop() {
delay(50); // Wait 50ms between pings (about 20 pings/sec). 29ms should be the shortest delay between pings.
Serial.print("Ping: ");
Serial.print(sonar.ping_cm()); // Send ping, get distance in cm and print result (0 = outside set distance range)
Serial.println("cm");
}
This system is useful because it minimizes the number of pins you use on the microcontroller. Result
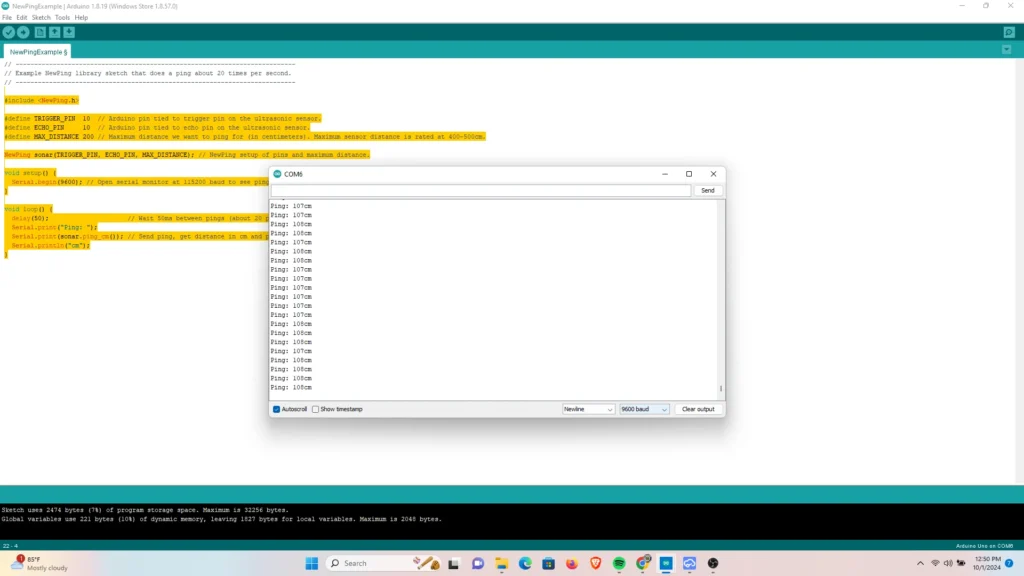
How to build a network of ultrasonic sensors using newping.h library
In this example, we will build a network of three ultrasonic sensors and print the distance they each measure. You can use more ultrasonic sensors and adjust the code and circuit to suit your design.
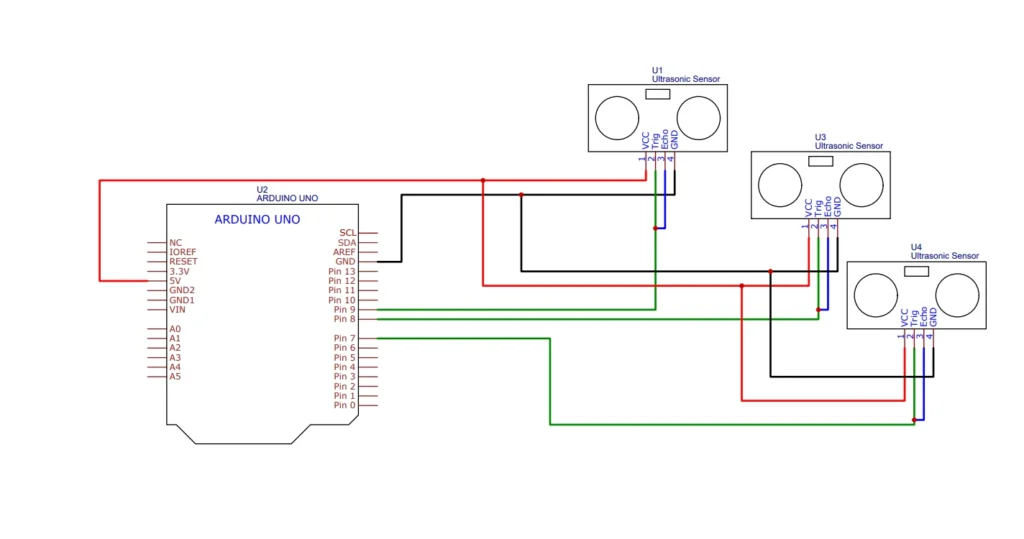
#include <NewPing.h>
#define MAX_DISTANCE 200
NewPing sonar1(7, 7, MAX_DISTANCE);
NewPing sonar2(8, 8, MAX_DISTANCE);
NewPing sonar3(9, 9, MAX_DISTANCE);
int distance[3];
int bcount = 3;
void setup(){
Serial.begin(9600);
}
void readDistance(){
distance[0]=sonar1.ping_cm();
if(distance[0]>200){
distance[0]=200;
}
delay(50);
distance[1]=sonar2.ping_cm();
if(distance[1]>200){
distance[1]=200;
}
delay(50);
distance[2]=sonar3.ping_cm();
if(distance[2]>200){
distance[2]=200;
}
delay(50);
}
void loop(){
for (int i=0; i<bcount; i++){
Serial.print(distance[i]);
Serial.print("\t");
}
Serial.println();
readDistance();
}
In the above code, we store the distance read by each ultrasonic sensor and store it in the array distance[3]. We used a for loop to print out each value in the serial monitor. Results below.
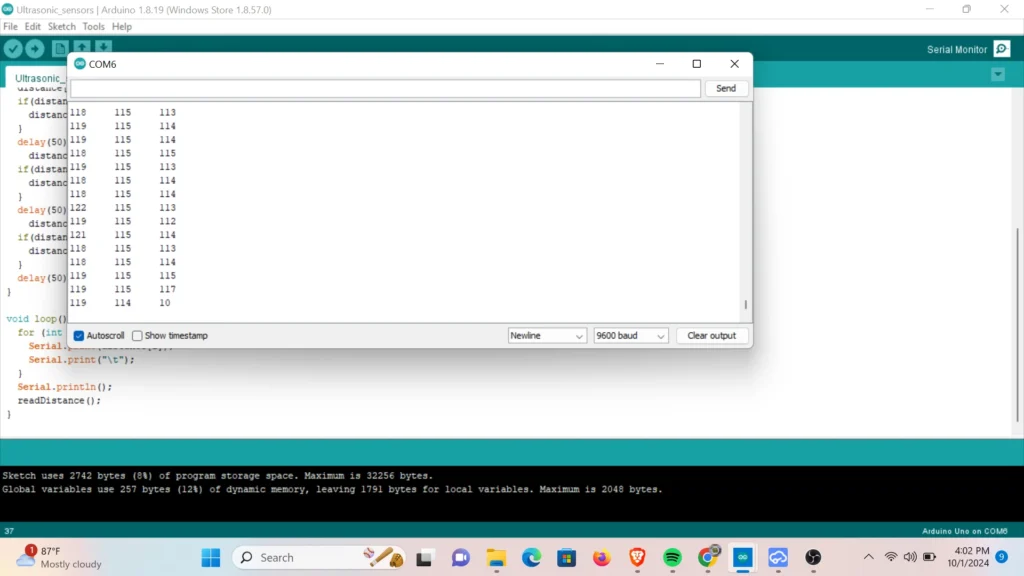
Images from example
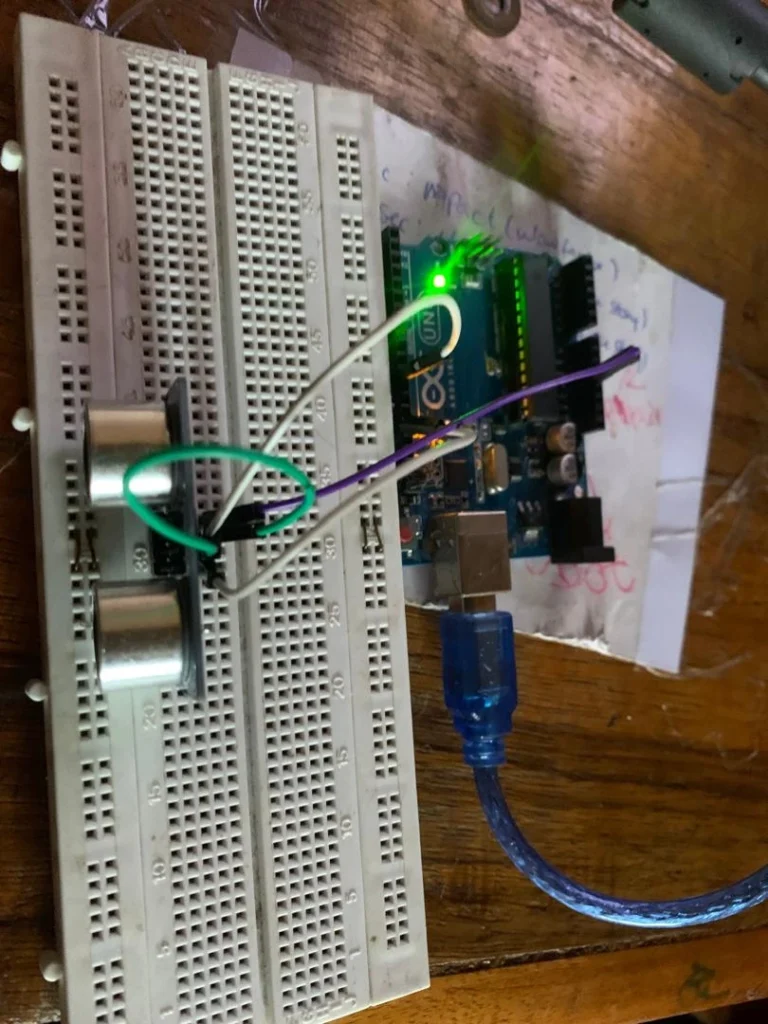
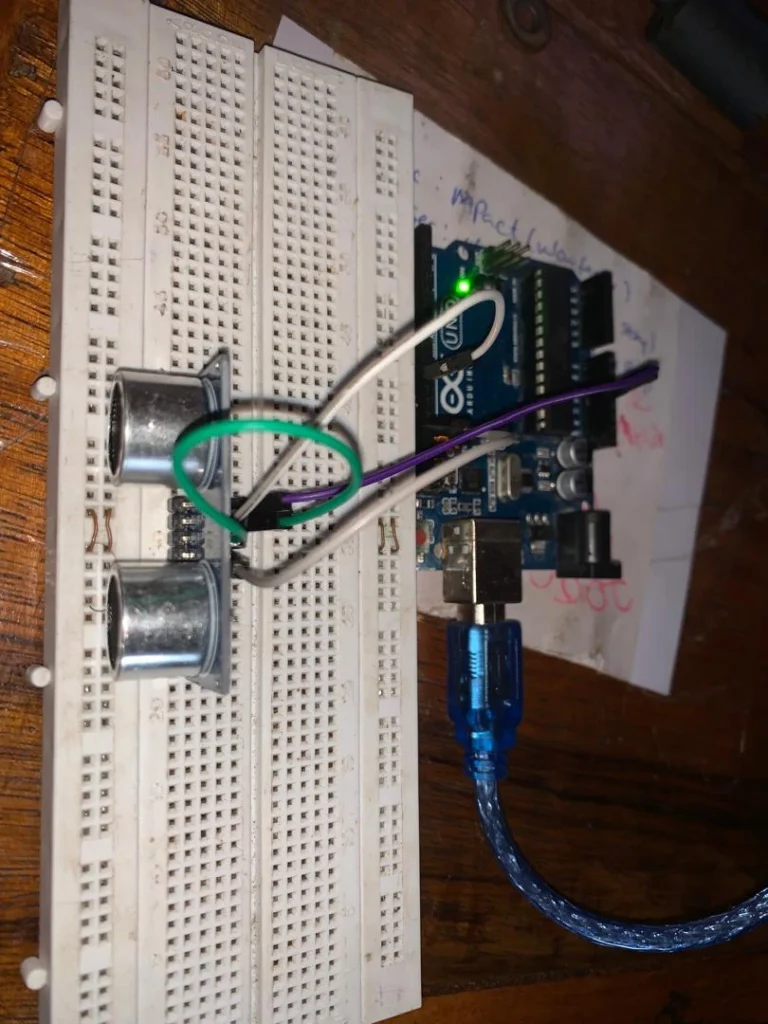
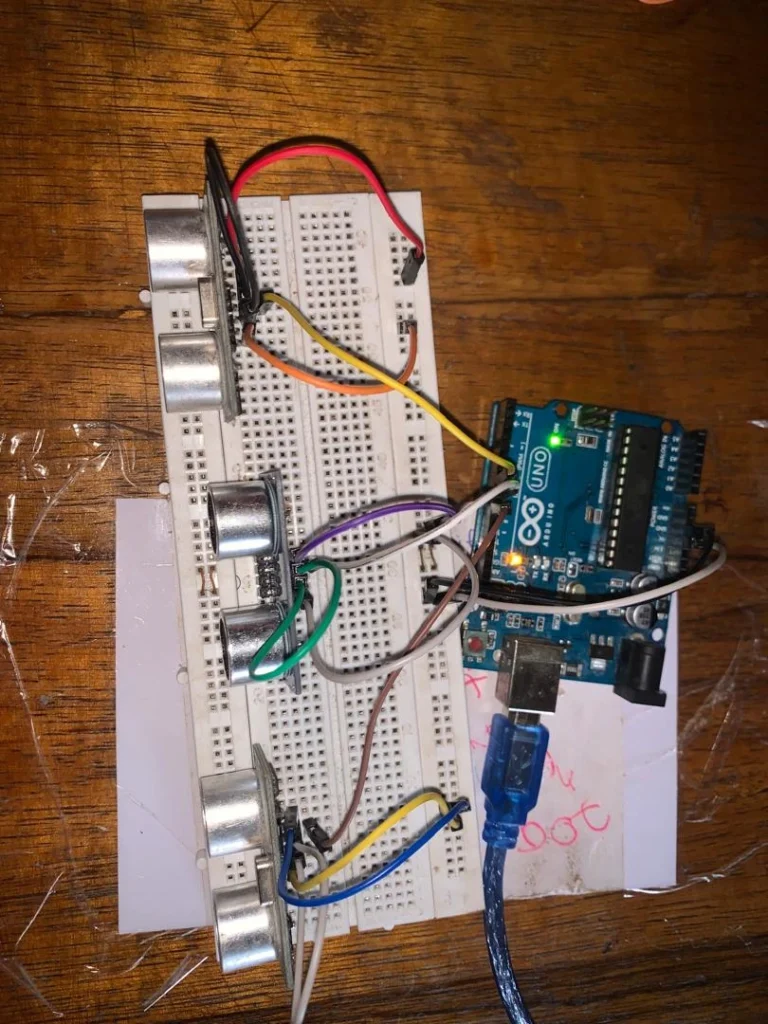
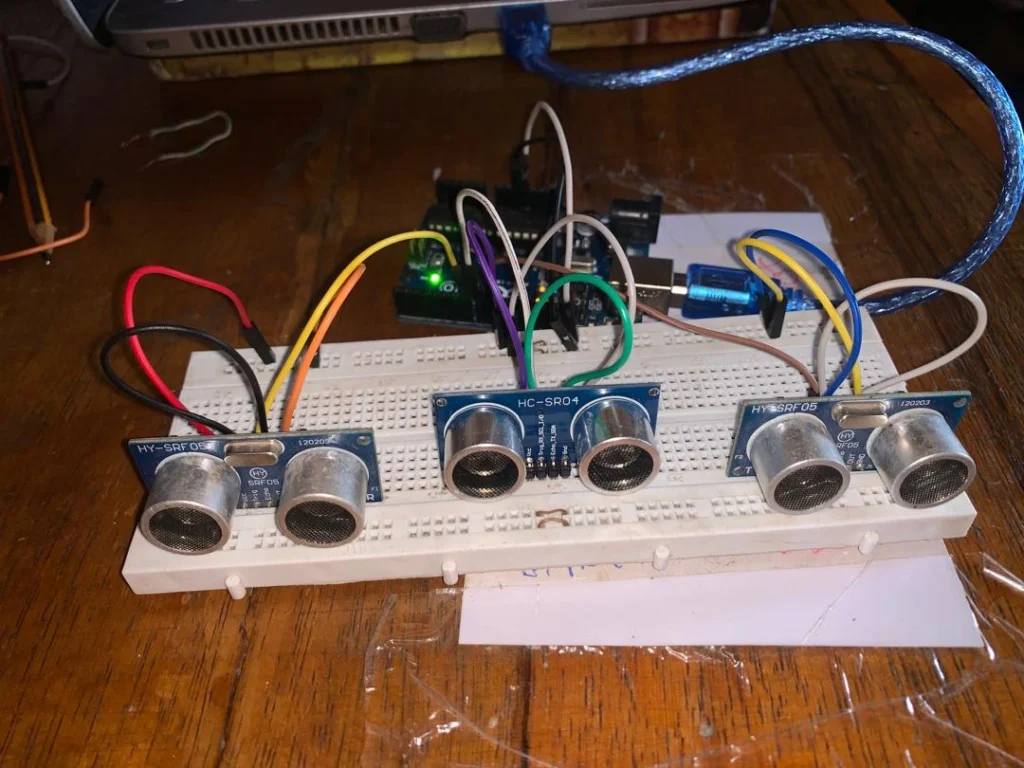
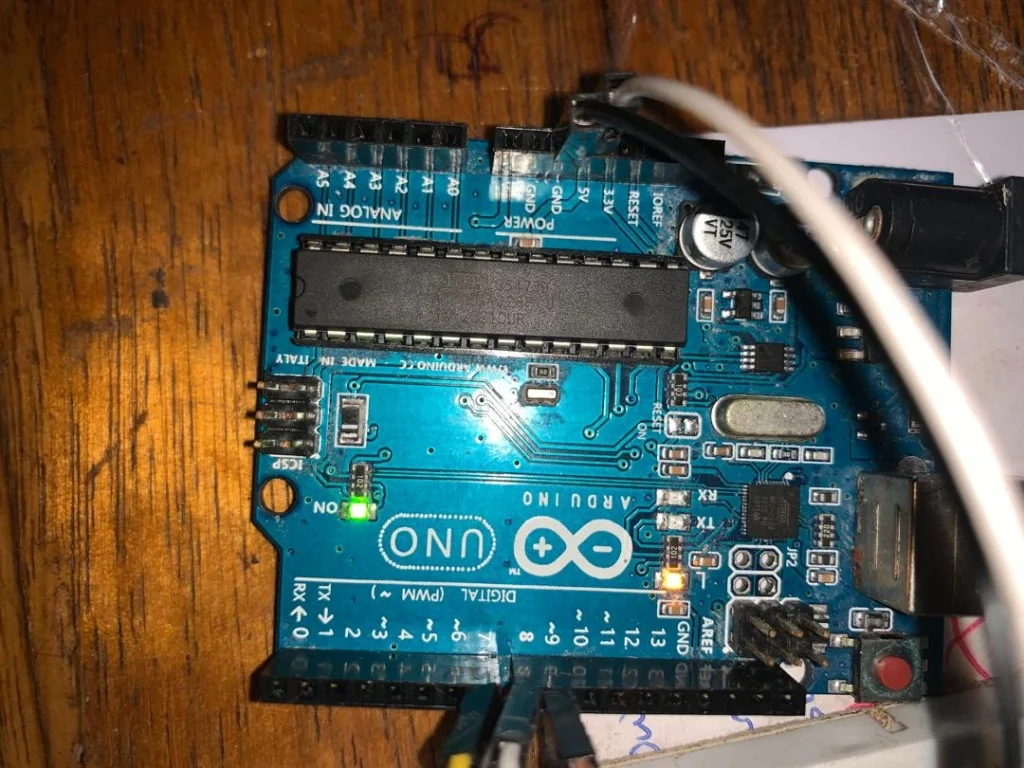
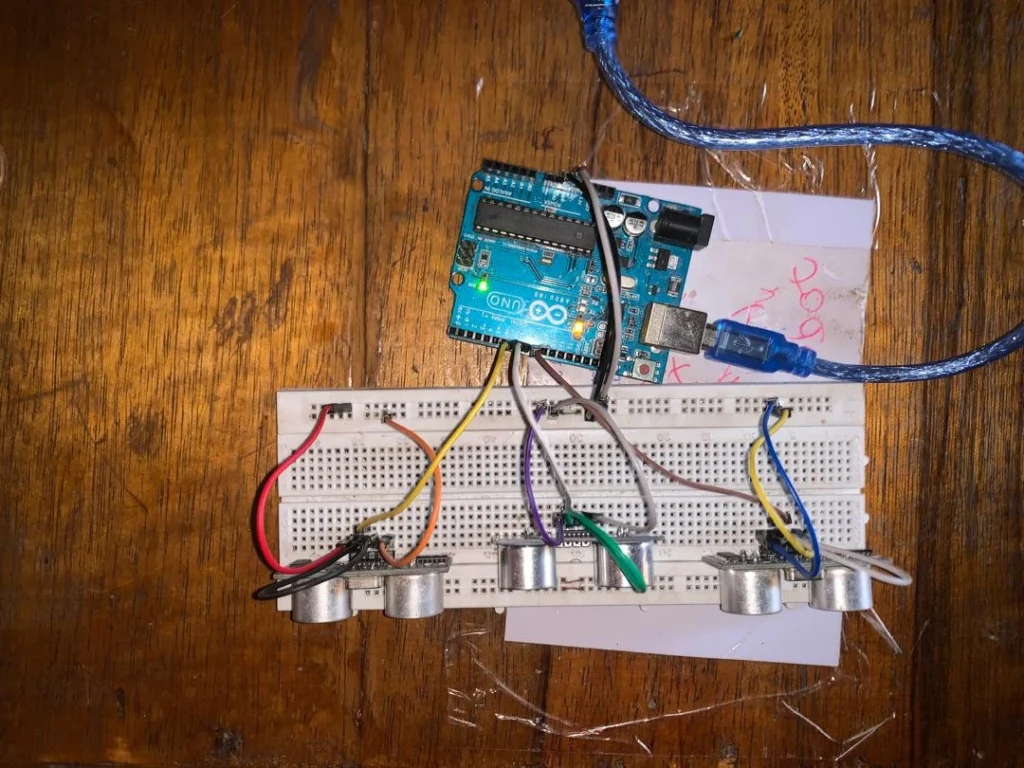